132. JAVA Program to solve Tower of Hanoi using recursion.
How does this program work?
- In this program you will learn about how to solve tower of hanoi problem using recursion in java.
Here is the code
//To solve Tower of Hanoi using recursion
import java.util.Scanner;public class Hanoi
{
public static void shift(int n, String startPole, String intermediatePole, String endPole)
{
if ( n == 0 )
{
return;
}shift(n - 1, startPole, endPole, intermediatePole);
System.out.println("Move \"" + n + "\" from" + startPole + " --> " + endPole);
shift(n - 1, intermediatePole, startPole, endPole);
}
public static void main(String[] args)
{
System.out.print("Enter number of discs:\n ");
Scanner obj = new Scanner(System.in);
int numberOfDiscs = obj.nextInt();
shift(numberOfDiscs, "Pole1", "Pole2", "Pole3");
}
}
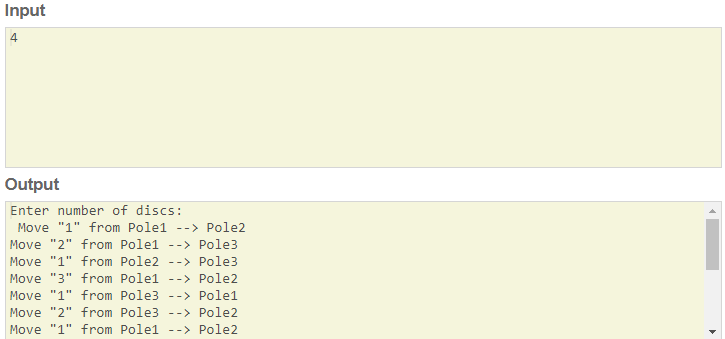