9. PHP Program to Calculate age in days.
How does this program work?
- In this program you will learn about how to calculate your age in days using PHP.
- This program requires the user to choose your date of birth.
- We will be calcualte age in days by followed by below code.
Here is the code
<html>
<head>
<title>PHP Program To find your age in days</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> Choose your DOB </td>
</tr>
<tr>
<td> <select name = "yy" >
<option value = ""> Year </option>
<?php
for($i = 1900; $i <= 2014; $i++)
{
echo "<option value = '$i'> $i </option>";
}
?>
</select>
<select name="mm">
<option value=""> Month </option>
<?php
for($i = 1; $i <= 12; $i++)
{
echo "<option value = '$i'> $i </option>";
}
?>
</select>
<select name="dd">
<option value=""> Date </option>
<?php
for($i = 1; $i <= 31; $i++)
{
echo "<option value = '$i'> $i </option>";
}
?>
< /select>
</td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/></td >
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$mm = $_POST['mm'];
$dd = $_POST['dd'];
$yy = $_POST['yy'];
$dob = $mm."/".$dd."/".$yy;
$arr = explode('/',$dob);
//$dateTs=date_default_timezone_set($dob);
$dateTs = strtotime($dob);
$now = strtotime('today');
if(sizeof($arr)!=3) die('Error: Please enter a valid date');
if(!checkdate($arr[0],$arr[1],$arr[2])) die('Please: Enter a valid dob');
if($dateTs >= $now) die('Enter a dob earlier than today');
$ageDays = floor(($now - $dateTs)/86400);
$ageYears = floor($ageDays/365);
$ageMonths = floor(($ageDays-($ageYears * 365))/30);
echo " You are aprox $ageYears years and $ageMonths months old ";
}
?>
</body>
</html>
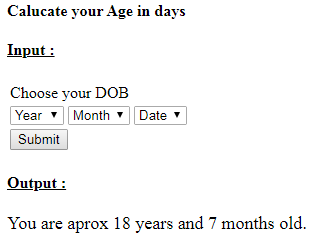
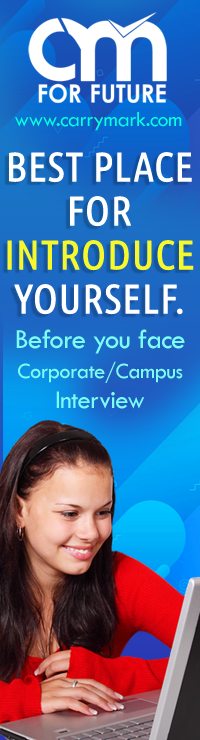