10. PHP Program to find Simple Interest and Compound Interest.
How does this program work?
- In this program you will learn about how to find Simple Interest and Compound Interest using PHP.
- This program requires the user input to enter the principle amount, time peroid and rate of interest.
- And then by using the given logic you will get the output.
Here is the code
<html>
<head>
<title>PHP Program To find Simple Interest and Compound Interest</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter principal"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter time period"/> </td>
</tr>
<tr>
<td> <input type="text" name="num3" value="" placeholder="Enter rate of interest"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$p = $_POST['num1'];
$t = $_POST['num2'];
$r = $_POST['num3'];
//Simple Interest formula
$si = $p * $t * $r/100;
echo "Simple interest = ".$si;
//Compound Interest formula
$amount = $p * pow((1 + $r/100),$t);
$ci = $amount - $p;
echo "Compound interest = ".$ci ;
return 0;
}
?>
</body>
</html>
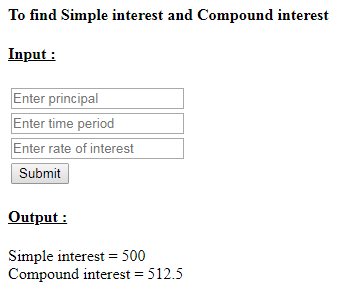
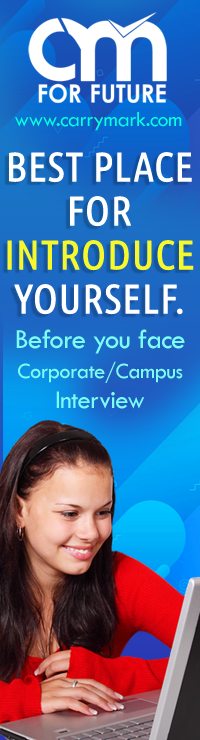