11. PHP Program to find Mechanical Energy of a particle is given by e=mgh+(1/2)mv^2.
How does this program work?
- In this program you will learn about how to calculate Mechanical Energy using PHP.
- This program requires the user inputs Mass ,displacement and velocity of an object.
- In this program First we calculate Potential Energy and Kinetic Energy.
- After that we will be find Mechanical energy by the combination of kinetic energy and potential energy.
Here is the code
<html>
<head>
<title>PHP Program To find Mechanical Energy of a particle</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter Mass of an object"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter displacement of an object"/> </td>
</tr>
<tr>
<td> <input type="text" name="num3" value="" placeholder="Enter velocity of an object"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$m = $_POST['num1'];
$h = $_POST['num2'];
$v = $_POST['num3'];
$P = $m*9.8*$h; //To calculate Potential energy(P.E = mgh)
$K = 0.5 * $m * $v * $v; //To calculate Kinetic energy(K.E = 1/2mv^2)
$Me = $P + $K; //To calculate Mechanical energy(M.E = P.E+K.E)
echo "Potential energy = ".$P."J" ;
echo "Kinetic energy = ".$K ."J";
echo "Mechanical energy = ".$Me."J";
return 0;
}
?>
</body>
</html>
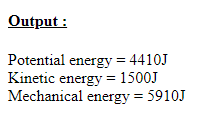
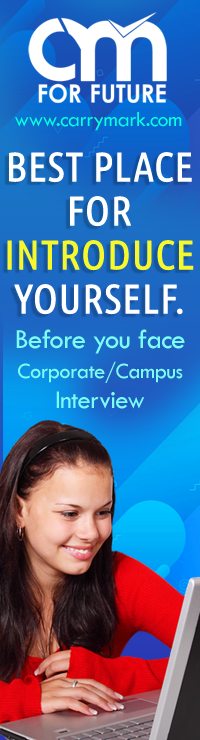