39. PHP Program to Check given Input is Vowel or Not using Switch Case.
How does this program work?
- In this program you are going to learn about how to Identify the given input is Vowel or Consonant using Switch Case in PHP.
Here is the code
<html>
<head>
<title>PHP Program To Identify given input is Vowel or Consonant using Switch Case</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter any alphabet"/></td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$ch = $_POST['num'];
switch($ch)
{
case 'a':
case 'e':
case 'i':
case 'o':
case 'u':
case 'A':
case 'E':
case 'I':
case 'O':
case 'U':
echo "Given letter ".$ch. " is Vowel" ;
break;
default:
echo "Given letter ".$ch." is Consonant" ;
}
return 0;
}
?>
</body>
</html>
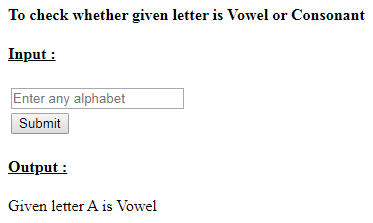
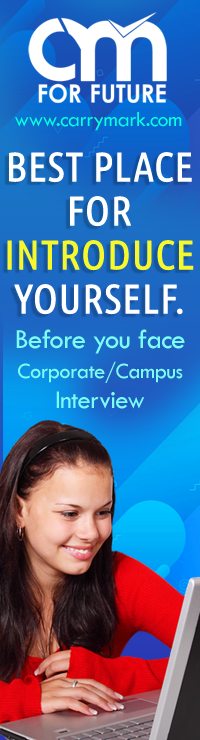