40. PHP Program to find the Biggest of two numbers using Switch Case.
How does this program work?
- In this program you are going to learn about how to find the biggest number among two numbers by using Switch Case in PHP.
- The integer entered by the user is stored in two variables a and b.
- After that compare two numbers, If a is greater than b then print a is biggest else print b is biggest using switch.
Here is the code
<html>
<head>
<title>PHP Program To Find biggest of two numbers using Switch Case</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter 1st number"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter 2nd number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$a = $_POST['num1'];
$b = $_POST['num2'];
echo "Given input is: "; //To print input
echo $a;
echo $b;
//If Expression ($a > $b ) will return either 0 or 1
switch($a > $b)
{
//If condition ($a>$b) is false
case 0:
echo " $b is a Big number " ;
break;
// If condition ($a>$b) is true
case 1:
echo " $a is a Big number " ;
break;
}
return 0;
}
?>
</body>
</html>
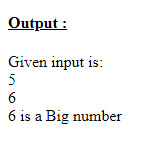
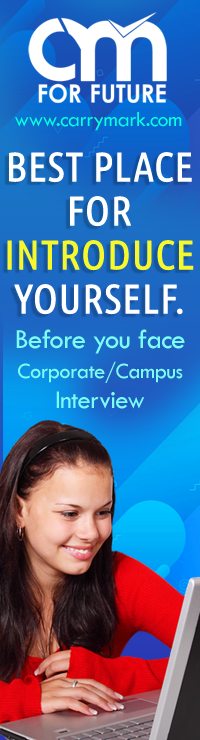