41. PHP Program to Perform Arithmetic Operations using Switch Case.
How does this program work?
- In this program we are going to learn about how to perform arithematic operations by using Switch Case in PHP.
- The integer entered by the user is stored in two variables a, b and also entered choice is stored in variable ch.
- By using switch case select one option then equivalent operation (Addtion, Subtraction, Multiplication, Division) can be performed by given logic.
Here is the code
<html>
<head>
<title>PHP Program To Calculate Arithmetic operations using Switch Case</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter 1st number"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter 2nd number"/> </td>
</tr>
<tr>
<td> <input type="text" name="option" value="" placeholder="Enter any option"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$a = $_POST['num1'];
$b = $_POST['num2'];
$ch = $_POST['option'];
echo "1 -> Addition<br>";
echo "2 -> Subtraction<br>";
echo "3 -> multiplication<br>";
echo "4 -> Divison<br>";
switch($ch)
{
case 1:
$r = $a+$b;
echo " Addition = ".$r ;
break;
case 2:
$r = $a-$b;
echo " Subtraction = ".$r ;
break;
case 3:
$r = $a*$b;
echo " Multiplication = ".$r ;
break;
case 4:
$r = $a/$b;
echo " Divison = ".$r ;
break;
default:
echo ("invalid option\n");
}
return 0;
}
?>
</body>
</html>
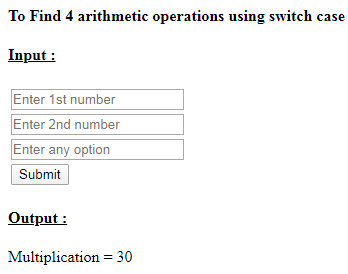
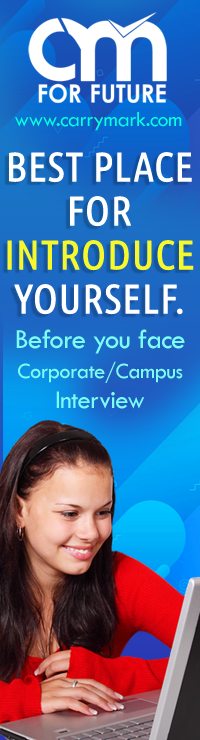