42. PHP Program to Count the number of notes available in given Amount.
How does this program work?
- In this program we are going to learn about how to Count the number of notes available in given amount using PHP.
- Here we are using array concept and find the number of different denominations that sum upto the given amount. Starting from the highest denomination note.
- We may assume that notes of values like 2000, 500, 200, 100, 50, 20, 10, 5, 1.
Here is the code
<html>
<head>
<title>PHP Program To Count the number of notes available in given amount</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter amount"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$amount = $_POST['num'];
$amount1 = $amount;
$notes = array(2000, 500, 200, 10, 50, 20, 10, 5, 1);
$noteCounter =array(0, 0, 0, 0, 0, 0, 0, 0, 0, 0);
for ($i = 0; $i < 9; $i++) // Count notes
{
if ($amount >= $notes[$i])
{
$noteCounter[$i] = intval($amount / $notes[$i]);
$amount = $amount - $noteCounter[$i] * $notes[$i];
}
}
echo "Amount is : ".$amount1; // Print given amount
echo ("Currency Count ->"); // Print notes
for ($i = 0; $i < 9; $i++)
{
if ($noteCounter[$i] != 0)
{
echo ($notes[$i] . " : " .$noteCounter[$i] );
}
}
return 0;
}
?>
</body>
</html>
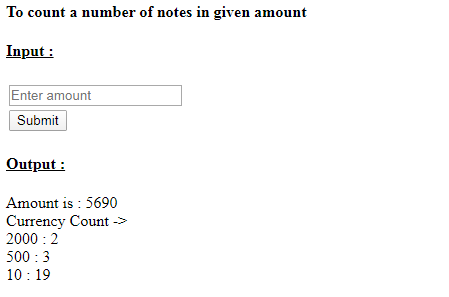
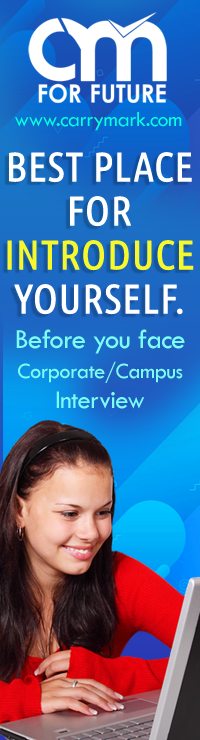