33. PHP Program to Check Middle digit of given 3 digit number is Equal to the Sum of Other Two digits or Not.
How does this program work?
- In this program you are going to learn about how to Check Middle digit of given 3 digit number is Equal to the Sum of Other Two digits or Not using PHP.
- Here First we claculate Last digit, First digit and their Sum.
- After that By using Mathematical Calculations like logarithm then compare Sum and middle digit using if condition and display result.
Here is the code
<html>
<head>
<title>PHP Program To Check middle digit is Equal to the Sum of Other Two digits or Not</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter any 3 digit number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$n = $_POST['num'];
$lastDigit = $n % 10; // Find last digit
$middle = ($n / 10) % 10 ; // Find middle digit
// Total number of digits - 1
$digits = (int)log10($n); // Find first digit
$firstDigit = (int)($n / pow(10, $digits));
$a = $lastDigit + $firstDigit;
echo "Given number is: ".$n;
if($a== $middle)
{
echo " Middle number is equal to sum of first digit and last digit ";
}
else
{
echo " Middle number is not equal to sum of first digit and last digit " ;
}
return 0;
}
?>
</body>
</html>
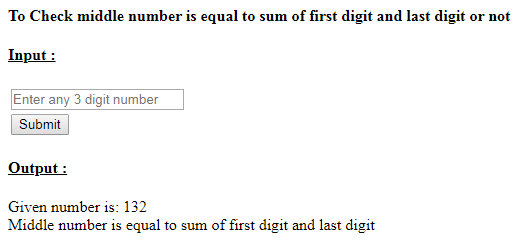
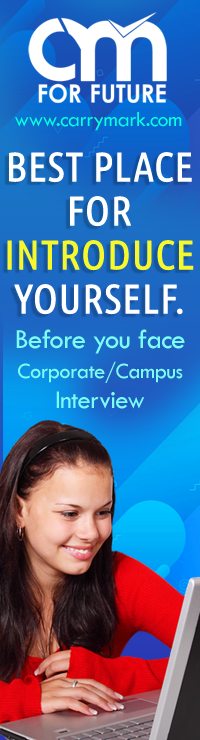