32. PHP Program to Calculate Monthly Income of a Person.
How does this program work?
- In this program you are going to learn about how to calculate Monthly Income of a Person folowing given Sales Commission Schedule using PHP.
- Here we are using if and nested if..else statements.
- After that By using the given Conditions Monthly income will be Calculated.
Here is the code
<html>
<head>
<title>PHP Program To calculate Monthly Income of a Person</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter Total sales of a person"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$a = $_POST['num'];
echo "Total sales of a person : ".$a;
if($a>50000)
{
$n = 16%$a;
$s = 375+$n;
echo ("Total Monthly sale income of a person is : ".$s);
}
else
if($a<50000 && $a>40000)
{
$n = 14%a;
$s = 370+$n;
echo ("Total Monthly sale income of a person is : ".$s);
}
else
if($a<40000 && $a>30000)
{
$n = 12%$a;
$s = 325+$n;
echo ("Total Monthly sale income of a person is : ".$s);
}
else
if($a<30000 && $a>20000)
{
$n = 9%$a;
$s = 300+$n;
echo ("Total Monthly sale income of a person is : ".$s);
}
else
if($a<20000 && $a>10000)
{
$n = 5%$a;
$s = 250+$n;
echo ("Total Monthly sale income of the person is : ".$s);
}
return 0;
}
?>
</body>
</html>
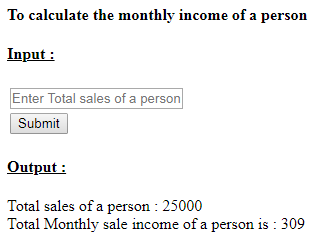
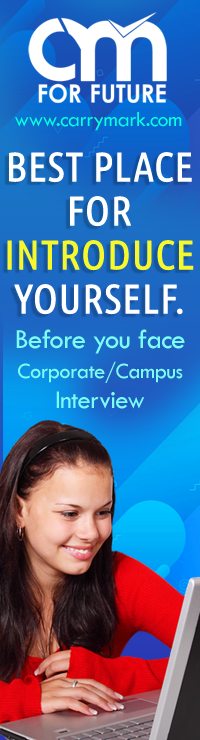