31. PHP Program to read three sides of a triangle and print the type of the triangle.
How does this program work?
- In this program you are going to learn about how to find the which type of the triangle using its three sides.
-
We can solve this problem by first calculating the side length and then classifying on comparing of side lengths.
- if all sides are equal, triangle will be equilateral.
- if any two sides are equal triangle will be Isosceles otherwise it will be Scalene.
- Now angle can be classified by Pythagoras theorem, if sum of square of two sides is equal to square of the third side, triangle will be right angle.
Here is the code
<html>
<head>
<title>PHP Program To read three sides of a triangle and print the type of the triangle</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter length of 1st side"/>
</td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter length of 2nd side"/>
</td>
</tr>
<tr>
<td> <input type="text" name="num3" value="" placeholder="Enter length of 3rd side"/>
</td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/>
</td> </tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$a = $_POST['num1'];
$b = $_POST['num2'];
$c = $_POST['num3'];
echo "Given sides : ".$a." ".$b." ".$c. "</br>";
if(($a*$a)+($b*$b)==($c*$c) || ($b*$b)+($c*$c)==($a*$a) || ($c*$c)+($a*$a)==($b*$b))
{
echo("Right angle triangle");
}
else
if(($a==$b) && ($b==$c))
{
echo("Equilateral triangle");
}
else
if(($a==$b) || ($b==$c) || ($c==$a))
{
echo("Isosceles triangle");
}
else
if(($a!=$b && $b!=$c && $c!=$a))
{
echo("Scalene triangle");
}
return 0;
}
?>
</body>
</html>
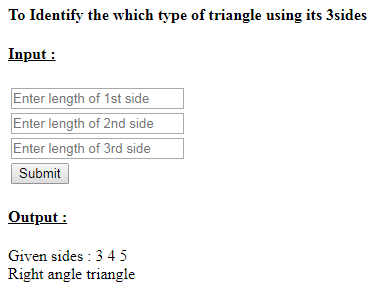
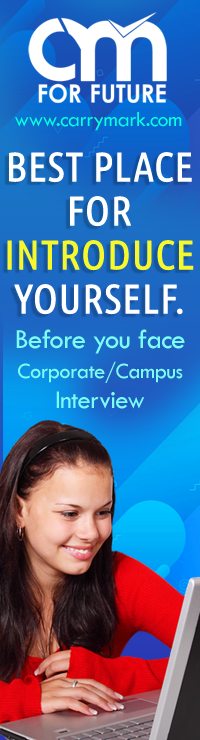