191. PHP Program to find the given number is Disarium number or Not.
How does this program work?
- In this program you are going to learn about to check if a number is Disarium number or not using PHP.
- A number is said to be the Disarium number, if sum of its digits powered with their respective positions is equal to the number itself.
- First find the length of a number and calculate the sum of digits powered with their respective position.
- After that check the condition followed by given logic then we get the Output.
Here is the code
<html>
<head>
<title>PHP Program To Check a given number is Disarium number or Not</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num" value="" placeholder="Enter a number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
//To calculate length of the given number
function calculateLength($n)
{
$length = 0;
while($n != 0)
{
$length = $length + 1;
$n = intval($n/10);
}
return $length;
}
if(isset($_POST['submit']))
{
$num =$_POST['num'];
//Makes a copy of the original number num
$n = $num;
$rem = $sum = 0;
$len = calculateLength($num);
//Calculates the sum of digits powered with their respective position
while($num > 0)
{
$rem = $num%10;
$sum = $sum + pow($rem,$len);
$num = intval($num/10);
$len--;
}
if($sum==$n)
{
echo $n. " is a disarium number";
}
else
{
echo $n. " is not a disarium number";
}
}
?>
</body>
</html>
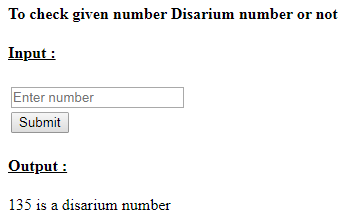
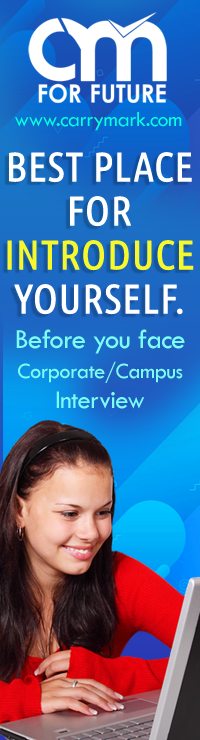