190. PHP Program to find neither bigger nor smaller out of given 3 numbers.
How does this program work?
- In this program you are going to learn about how to find neither big nor small out of given 3 numbers using PHP.
- The integer entered by the user is stored in variables n1, n1 and n3.
- After that we can find the middle number by using if conditions.
Here is the code
<html>
<head>
<title>PHP Program To find neither big nor small out of given 3 numbers</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter 1st number"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter 2nd number"/> </td>
</tr>
<tr>
<td> <input type="text" name="num3" value="" placeholder="Enter 3rd number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
function middle($a, $b, $c)
{
if( $b>$a && $a>$c || $c>$a && $a>$b )
{
echo ("$a is middle number");
}
//Checking for b is middle number or not
if( $a>$b && $b>$c || $c>$b && $b>$a )
{
echo("$b is middle number");
}
//Checking for c is middle number or not
if( $a>$c && $c>$b || $b>$c && $c>$a )
{
echo("$c is middle number");
}
}
if(isset($_POST['submit']))
{
$n1 = $_POST[ 'num1' ];
$n2 = $_POST[ 'num2'];
$n3 = $_POST[ 'num3'];
echo "First number = ".$n1."</br>";
echo "Second number = ".$n2."</br>";
echo "Third number = ".$n3."</br>";
middle($n1, $n2, $n3);
}
?>
</body>
</html>
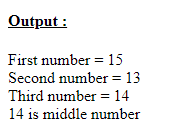
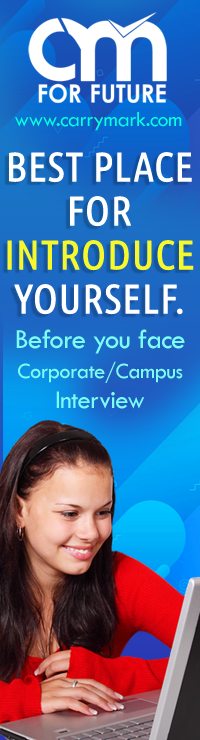