200. PHP Program to find total number of characters in a string.
How does this program work?
- This program is used to find a character and replace with another character using PHP.
- Define and initialize a variable count to 0.
- Iterate through the string till the end and for each character except spaces, increment the count by 1.
- To avoid counting the spaces check the condition i.e. string[i] != ' '.
Here is the code
<html>
<head>
<title>PHP Program To find total number of characters in a string</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="str" value="" placeholder="Enter string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$string = $_POST['str'];
$count = 0;
//Counts each character except space
for($i = 0; $i < strlen($string); $i++)
{
if($string[$i] != ' ')
$count++;
}
echo "Given String : ".$string."<br>";
//Displays the total number of characters present in the given string
echo "Total number of characters in a string: " . $count;
}
?>
</body>
</html>
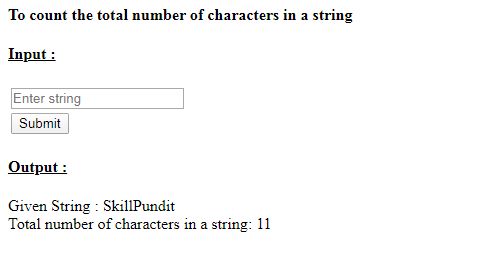
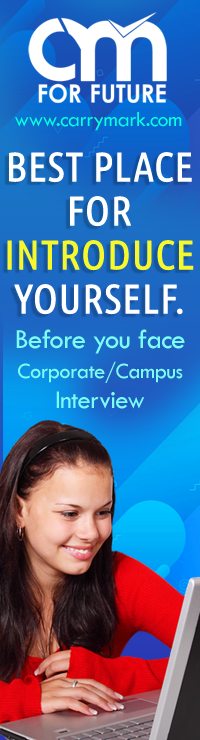