199. PHP Program to find the Largest and Smallest word in a String.
How does this program work?
- This program is used to find the Largest and Smallest word in a String using PHP.
- First convert the string into lower case and add an extra space at the end.
- Declare and Initialize variable small and large with first word of array.
- check if the length of word is less than small, then that word will be stored in small Otherwise If the length of word is greater than largethen that word will be stored in large
- Finally, we can find largest and smallest word.
Here is the code
<html>
<head>
<title>PHP Program To find largest and smallest word in a string </title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="string" value="" placeholder="Enter string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$string = $_POST['string'];
$word = "";
$words =array();
//Add extra space after string to get the last word in the given string
$string = $string . " ";
for($i = 0; $i < strlen($string); $i++)
{
//Split the string into words
if($string[$i] != ' ')
{
$word = $word . $string[$i];
}
else
{
//Add word to string array words
array_push($words, $word);
//Make word an empty string
$word = "";
}
}
//Initialize small and large with first word in the string
$small = $large = $words[0];
//Determine smallest and largest word in the string
for($k = 0; $k < count($words); $k++)
{
//To find the lengths and compare them
if(strlen($small) > strlen($words[$k]))
$small = $words[$k];
//To find the lengths and compare them
if(strlen($large) < strlen($words[$k]))
$large =$words[$k];
}
echo("Given string: " . $string."<br>");
echo("Smallest word: " . $small."<br>");
echo("Largest word: " . $large."<br>");
}
?>
</body>
</html>
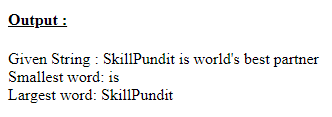
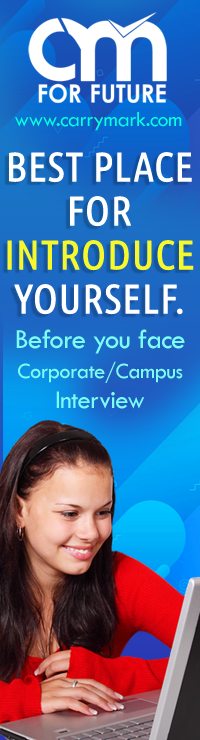