198. PHP Program to find the frequency of characters.
How does this program work?
- This program is used to find the frequency of characters of given string using PHP.
- Define an array freq with the same size of the string.
- Two loops will be used to count the frequency of each character. Outer loop will be used to select a character and initialize element at corresponding index in array freq with 1.
- Inner loop will compare the selected character with remaining characters present in the string.
- If a match found, increment element in freq by 1 and set the duplicates of selected character by '0' to mark them as visited.
- Finally, display the character and their corresponding frequencies.
Here is the code
<html>
<head>
<title>PHP Program To find the frequency of characters</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="name1" value="" placeholder="Enter string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$str = $_POST['name1'];
$freq = array();
//To find length of the given string
for($i = 0; $i < strlen($str); $i++)
{
array_push($freq, 1);
for($j = $i+1; $j < strlen($str); $j++)
{
if($str[$i] == $str[$j])
{
$freq[$i]++;
//Set $str[$j] to 0 to avoid printing visited character
$str[$j] ='0';
}
}
}
//Displays the each character and their corresponding frequency
echo ("Characters and their corresponding frequencies <br>");
for($i = 0; $i < count($freq); $i++)
{
if($str[$i] != ' ' && $str[$i] != '0')
{
echo($str[$i] . "-" . $freq[$i]);
echo("<br>");
}
}
}
?>
</body>
</html>
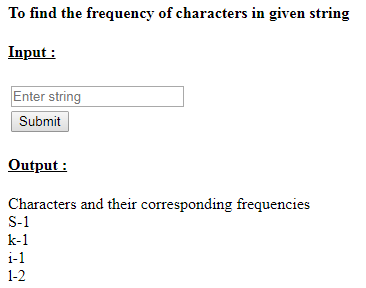
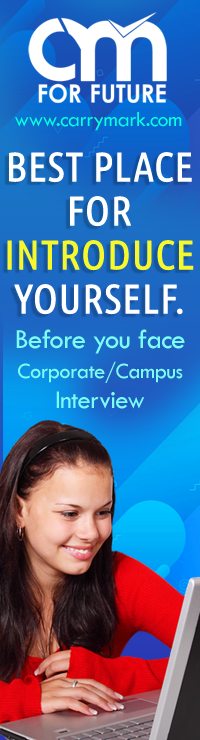