76. PHP Program to Display frequency of each element from array.
How does this program work?
- This program is used to find frequency of each element in given array using PHP.
- In this program we need to count the occurrence of each element present in the array.
- Declare two arrays one will be store elements, and another(fr) will be store the frequencies of elements present in an array.
- And variable check will initialize with -1, because it helps us to avoid counting the same element again in the given array.
Here is the code
<html>
<head>
<title>PHP Program To display frequency table of given array elements</title>
</head>
<body>
<?php
// Initialize array of elements
$a = array (54,85,92,54,16,92,48);
//If array will store frequencies of element
$fr = array_fill(0, count($a), 0);
$check = -1;
for($i = 0; $i < count($a); $i++)
{
$count = 1;
for($j = $i+1; $j < count($a); $j++)
{
if($a[$i] == $a[$j]) // If duplicate element is found increment count
{
$count++;
//To avoid counting same element again
$fr[$j] = $check;
}
} if($fr[$i] != $check) //If element is not repeated count will be same
$fr[$i] =$count;
}
echo("The Array Elements are: ");
foreach( $a as $b )
{
echo $b." ";
}
//Displays the frequency of each element in given array
echo (" frequency of given array elements: " );
for( $i = 0; $i < count($fr); $i++)
{
if($fr[$i] != $check)
{
echo( $a[$i] ." occurs " );
echo( $fr[$i]." times.");
echo(" ");
}
}
return 0;
?>
</body>
</html>
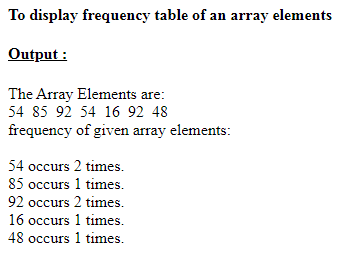
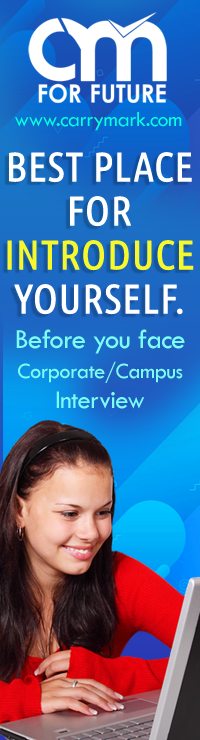