75. PHP Program to find the Second Biggest number from the given n numbers.
How does this program work?
- This program is used to find the Second Biggest number from the given N numbers using PHP.
- Each element is compared with the next element, If the element is greater than the other in comparison then the elements can be swapped.
- After doing this for each element of the given array, then we get the Output.
Here is the code
<html>
<head>
<title>PHP Program To find the Second biggest from the given n numbers</title>
</head>
<body>
<?php
function secondlarge($arr, $arr_size)
{
If ($arr_size < 2) // It should be more than 2 elements
{
echo(" Invalid Input ");
return;
}
$first = $second = 0;
for ($i = 0; $i < $arr_size ; $i++)
{
//First element is greater than current element then first and second elements can be change
if ($arr[$i] > $first)
{
$second = $first;
$first = $arr [$i];
}
//To check current element is in between first and second
else if ( $arr[$i] > $second && $arr[$i] != $first )
// Then second element can be update
$second = $arr[$i];
}
if ( $second == 0 )
echo ("</br> There is no second largest element\n" );
else echo ("</br >The second largest element is " . $second . "\n" );
}
$arr = array (65,97,81,10,26);
$n = sizeof($arr);
echo "Given numbers : "."</br>";
foreach($arr as $value)
{
echo $value." ";
}
secondlarge($arr, $n);
return 0;
?>
</body>
</html>
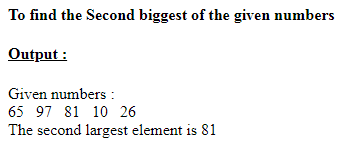
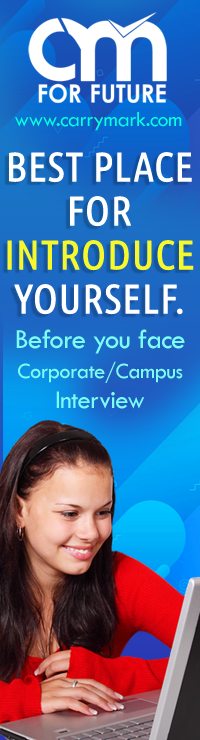