74. PHP Program to find LCM of given N numbers.
How does this program work?
- This program is used to find LCM of given N numbers using PHP.
- LCM (Least Common Multiple) of given numbers is the smallest number which can be divided by given numbers.
Here is the code
<html>
<head>
<title>PHP Program To find LCM of given N numbers</title>
</head>
<body>
<?php
function gcd($a, $b)
{
if ($b == 0)
return $a;
return gcd($b, $a % $b);
}
//To find LCM of array element
function lcmofn($arr, $n)
{
// Initialize result
$ans = $arr[0];
// Ans contains LCM of arr[0], ..arr[i] after i'th iteration.
for ($i = 1; $i < $n; $i++)
$ans = ((($arr[$i] * $ans)) / (gcd($arr[$i], $ans)));
return $ans;
}
$arr = array(2, 5, 30, 9, 14 );
$n = sizeof($arr);
echo "Given numbers : ";
foreach($arr as $value)
{
echo $value." ";
}
echo " LCM of given numbers are: ".(lcmofn($arr, $n));
return 0;
?>
</body>
</html>
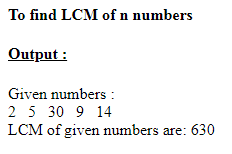
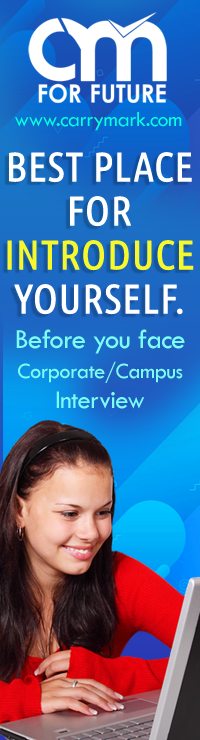