73. PHP Program to find GCD of given N numbers.
How does this program work?
- This program is used to find GCD or HCF of given N numbers using PHP.
- GCD or HCF (Highest Common Factor) of numbers is the largest number that divides by them.
Here is the code
<html>
<head>
<title>PHP Program To find GCD of given N numbers</title>
</head>
<body>
<?php
function gcd( $a, $b)
{
if ($a == 0)
return $b;
return gcd($b % $a, $a);
}
// Function to find gcd of array of numbers
function gcdofn($arr, $n)
{
$result = $arr[0];
for ($i = 1; $i < $n; $i++)
$result = gcd($arr[$i], $result);
return $result;
}
echo "Given numbers : ";
$arr = array( 25, 400, 65, 80, 50 );
foreach($arr as $value)
{
echo $value." ";
}
$n = sizeof($arr);
echo "GCD of given numbers is = ".(gcdofn($arr, $n));
return 0;
?>
</body>
</html>
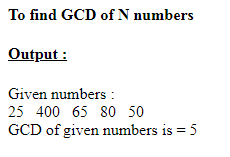
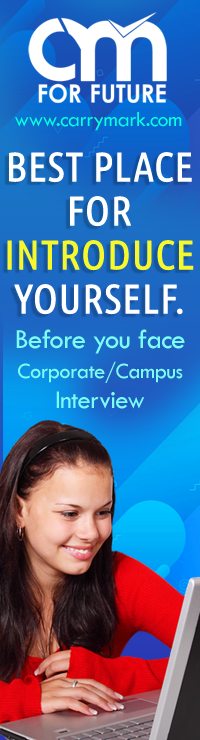