16. PHP Program to find the Distance between given two points.
How does this program work?
- In this program you will learn about how to find the distance between two points by using given X, Y co-odinates.
- First we need to store user entered values in x1, y1 and x2, y2.
- After that by using the given formula we can get the distance between two points by using PHP.
Here is the code
<html>
<head>
<title>PHP Program To find the distance between two points</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter x1 value"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter y1 value"/> </td>
</tr>
<tr>
<td> <input type="text" name="num3" value="" placeholder="Enter x2 value"/>
</td>
</tr>
<tr>
<td> <input type="text" name="num4" value="" placeholder="Enter y2 value"/>
</td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/>
</td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$x1 = $_POST['num1'];
$y1 = $_POST['num2'];
$x2 = $_POST['num3'];
$y2 = $_POST['num4'];
//Distance between two points (D = sqrt((x2-x1)^2 + (y2-y1)^2))
$x = $x2 - $x1;
$y = $y2 - $y1;
$distance = sqrt($x*$x + $y*$y);
$precision = 4;
echo"Distance between two points is: " .number_format($distance, $precision);
return 0;
}
?>
</body>
</html>
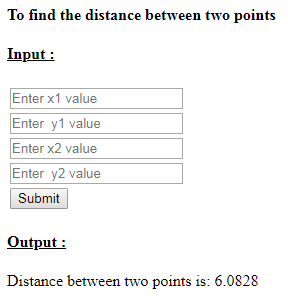
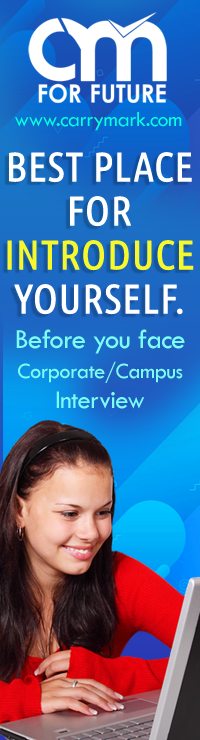