15. PHP Program to Interchange the contents of two variables without using temporary variable.
How does this program work?
- In this program you will learn about how to interchange the contents of two variables without using temperory variable.
- Here we take only two varibles A and B and the content will be stored in these two variable.
- After that by using given logic we can get the output using PHP.
Here is the code
<html>
<head>
<title>PHP Program To Interchanging two numbers without using Temporary variable</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter 1st number"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter 2nd number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit']))
{
$x = $_POST['num1'];
$y = $_POST['num2'];
echo "Before interchanging the values are: ";
echo "x = " .$x;
echo"y = " .$y;
$x = $x+$y;
$y = $x-$y;
$x = $x-$y;
echo "After interchanging the values are: ";
echo "x = " .$x;
echo "y = " .$y;
return 0;
}
?>
</body>
</html>
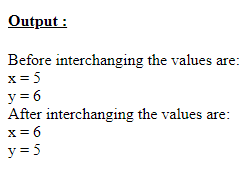
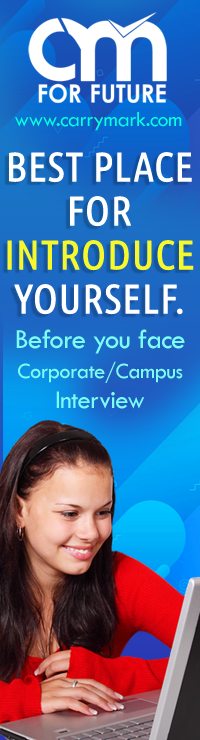