53. PHP Program to find the Biggest of the given numbers.
How does this program work?
- In this program we are going to learn about how to find the biggest of the given numbers using PHP.
- Declare big variable and initialize with 0.
- Each element is compared with the next element, If the element is greater than the other in comparison then that element can be stored in big variable.
- After doing this for each element of the given array, then we get the biggest number of given numbers.
Here is the code
<html>
<head>
<title>PHP Program To find the biggest of the given numbers</title>
</head>
<body>
<?php
$a =array(55, 96, 84, 64, 155);
$big = 0; //Initially we declare biggest number is 0
$c = count($a);
echo "Given numbers : ";
foreach($a as $value)
{
echo $value." ";
}
for($i = 0; $i < $c; $i++)
{
if ($a[$i] > $big)
//If biggest number is greater than a[0] element then value of a[0] stored in big variable
{
$big = $a[$i];
}
}
echo " Biggest of the given numbers is: ".$big;
return 0;
?>
</body>
</html>
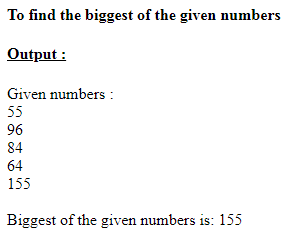
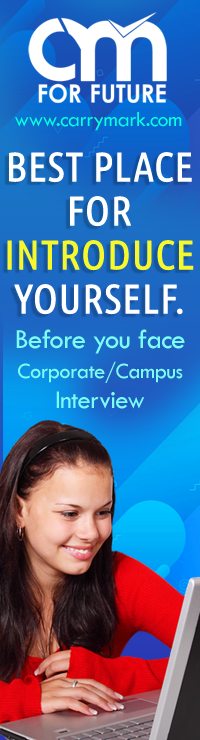