54. PHP Program to find the Second Smallest of the given numbers.
How does this program work?
- In this program we are going to learn about how to find the second smallest of the given numbers using PHP.
- Each element is compared with the next element, If the element is less than the other in comparison then the elements can be swapped.
- After doing this for each element of the given array, then we get the Output.
Here is the code
<html>
<head>
<title>PHP Program To find the second smallest of the given numbers</title>
</head>
<body>
<?php
$arr =array(56,23,49,62,95);
$n = count($arr);
echo "Elements : ";
foreach($arr as $value) //To print array elements
{
echo $value." ";
}
if ($arr < 2)
{
echo(" Invalid Input ");
return 0;
}
$first = $second = $arr;
for ($i = 0; $i < $n ; $i ++)
{
// If current element is smaller than first then update both first and second
if ($arr[$i] < $first) {
$second = $first;
$first =$arr[$i];
}
// If arr[i] is in between first and second then update second
else if ($arr[$i] < $second && $arr[$i] != $first)
$second = $arr[$i];
}
if ($second == $arr)
echo("There is no second smallest element\n");
else
echo "The Second Smallest element is ". $second;
return 0;
?>
</body>
</html>
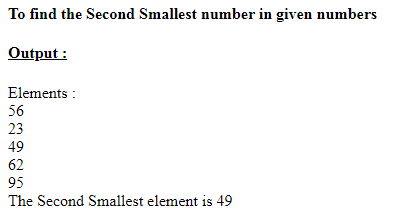
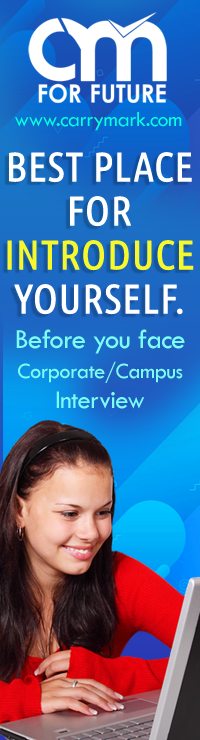