145. PHP Program to find the Fibonacci series of a given number using recursion.
How does this program work?
- This program is used to find the Fibonacci series of a given number using recursion in PHP.
- Fibonacci series means the previous two elements are added to get the next element starting with 0 and 1.
- First we initializing first and second number as 0 and 1, and print them.
- The third number will be the sum of the first two numbers by using loop.
- By using Recursion method we can find the fibonacci series of a given number.
Here is the code
<html>
<head>
<title>PHP Program To find the Fibonacci series of a given number</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter a number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
function Fibonacci($number) {
// if and else if to generate first two numbers
if ($number == 0)
return 0;
else if ($number == 1)
return 1;
// Recursive Call to get the upcoming numbers
else
return (Fibonacci($number-1) + Fibonacci($number-2));
}
if(isset($_POST['submit'])) {
$n = $_POST['num1'];
echo "Fibonacci series of $n is :"."
";
for ($counter = 0; $counter < $n; $counter++)
{
echo Fibonacci($counter),' ';
}
return 0;
}
?>
</body>
</html>
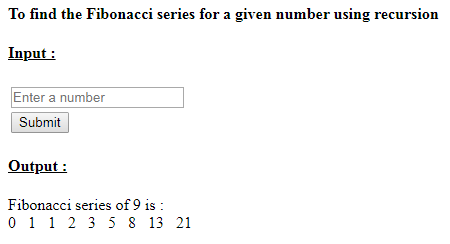
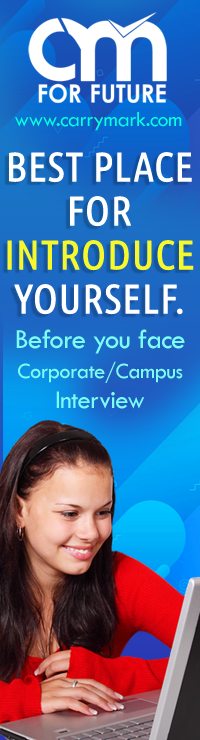