146. PHP Program to find GCD of N numbers using recursion.
How does this program work?
- This program is used to find GCD of N numbers using recursion in PHP.
- GCD or HCF (Highest Common Factor) of numbers is the largest number that divides by them.
Here is the code
<html>
<head>
<title>PHP Program To find GCD of N numbers recursively</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="num1" value="" placeholder="Enter 1st number"/> </td>
</tr>
<tr>
<td> <input type="text" name="num2" value="" placeholder="Enter 2nd number"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
function gcd($a, $b)
{
// Everything divides 0
if ($a == 0)
return $b;
if ($b == 0)
return $a;
// base case
if($a == $b)
return $a ;
// a is greater
if($a > $b)
return gcd( $a-$b , $b ) ;
return gcd( $a , $b-$a ) ;
}
if(isset($_POST[ 'submit'])) {
$a = $_POST[ 'num1'];
$b = $_POST[ 'num2'];
echo "GCD of $a and $b is ", gcd($a , $b) ;
return 0;
}
?>
</body>
</html>
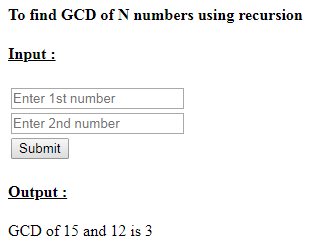
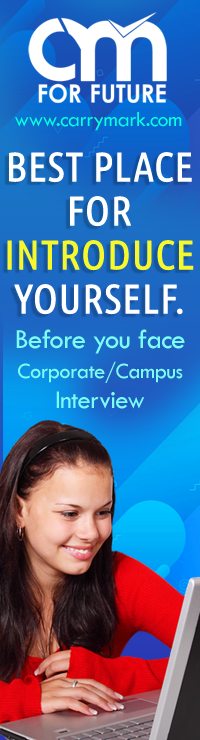