134. PHP Program to find Row sum and Column sum of a given matrix.
How does this program work?
- In this program we are going to learn about how to find Row sum and Column sum of given matrix using PHP.
- Elements can be stored in a variable using arrays. Declare variable sumRow and sumCol, and Initialize with 0.
- After that by using the forloop condition we find the Rowsum and Columnsum of given matrix.
Here is the code
<html>
<head>
<title>PHP Program To find Row sum and Column sum of given matrix</title>
</head>
<body>
<?php
//Initialize matrix a
$a = array
(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
//Calculates number of rows and columns present in given matrix
$rows = count($a);
$cols = count($a[0]);
echo("Original matrix is:</br>"); // To print Original matrix
for($i = 0; $i < $rows; $i++) {
for($j = 0; $j < $cols; $j++) {
echo ($a[$i][$j] . " ");
}
echo("</br>" );
}
echo "Row sum and Column sum of a matrix : "."<br>";
//Calculates sum of each row of given matrix
for($i = 0; $i < $rows; $i++) {
$sumRow = 0;
for($j = 0; $j < $cols; $j++) {
$sumRow = $sumRow + $a[$i][$j];
}
echo("Sum of " . ($i+1) ." row: " . $sumRow);
echo("</br>");
}
//Calculates sum of each column of given matrix
for( $i = 0; $i < $cols; $i++) {
$sumCol = 0;
for($j = 0; $j < $rows; $j++) {
$sumCol = $sumCol + $a[$j][$i];
}
echo("Sum of " . ($i+1) . " column: " . $sumCol);
echo("</br>");
}
?>
</body>
</html>
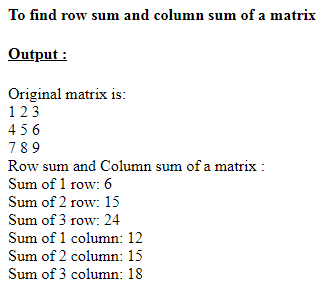
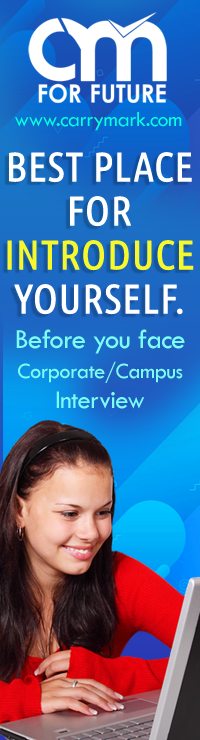