135. PHP Program to find Upper and Lower triangular of a given matrix.
How does this program work?
- In this program we are going to learn about how to find Upper and Lower trianglular of a given matrix using PHP.
- Elements can be stroed in variable using arrays.
- After that by using the forloop condition we find the Upper elements and Lower elements of given matrix.
Here is the code
<html>
<head>
<title>PHP Program To find Upper and Lower triangular of a given matrix</title>
</head>
<body>
<?php
function lower($a)
{
// To calculates the no.of rows and columns of given matrix
$rows = count($a);
$cols = count($a[0]);
if($rows != $cols)
{
echo("Matrix should be a square matrix</br>");
}
else {
// To convert given matrix into lower triangular matrix
echo( "Lower triangular matrix: </br>");
for($i = 0; $i < $rows; $i++)
{
for($j = 0; $j < $cols; $j++)
{
if($j > $i)
echo("0 ");
else
echo($a[$i][$j] . " ");
}
echo("</br>");
}
}
}
function upper($a)
{
// To calculates the no.of rows and columns of given matrix
$rows = count($a);
$cols = count($a[0]);
if($rows != $cols)
{
echo("Matrix should be a square matrix");
}
else {
//To convert given matrix into upper triangular matrix
echo("Upper triangular matrix: </br>");
for($i = 0; $i < $rows; $i++) {
for($j = 0; $j < $cols; $j++) {
if($i > $j)
echo("0 ");
else
echo($a[$i][$j] . " ");
}
echo("</br>");
}
}
}
// Elements of matrix a
$a = array
(
array(1, 2, 3),
array(8, 6, 4),
array(4, 5, 6)
);
echo lower($a);
echo upper($a);
?>
</body>
</html>
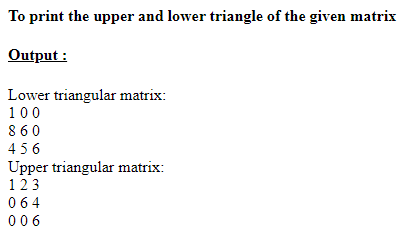
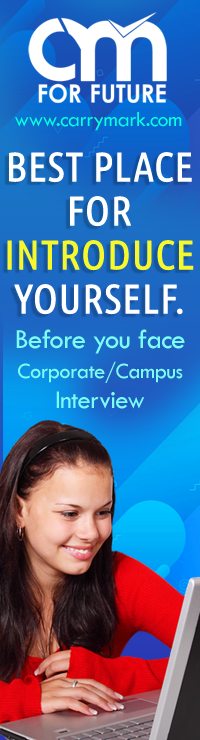