141. PHP Program to Find the Saddle point of the given matrix.
How does this program work?
- In this program we are going to learn about how to find the Saddle point of the matrix using PHP.
- Saddle point means minimum element in row is equal to the maximum element in column of a matrix.
- Find the minimum element in its row and maximum element in its column.
- Check if row minimum element and column maximum element. If it is equal then print saddle point, otherwise no saddle point present in given matrix
Here is the code
<html>
<head>
<title>PHP Program To find the Saddle point of the given matrix </title>
</head>
<body>
<?php
// Elements of matrix a
$a = array
(
array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9)
);
$n = count($a);
// To print original matrix
echo("Original matrix is: <br>");
for($i = 0; $i < $n; $i++) {
for($j = 0; $j < $n; $j++) {
echo ($a[$i][$j] . " ");
}
echo("<br>");
}
for($i = 0; $i< $n; $i++)
{
$min_row = $a[$i][0];
$col_ind = 0;
for ( $j = 1; $j < $n; $j++)
{
if ( $min_row > $a[$i][$j])
{
$min_row = $a[$i][$j];
$col_ind = $j;
}
}
// Check if the minimum element of row is equal to the maximum element of column or not
$k;
for ($k = 0; $k < $n; $k++)
if ($min_row < $a[$k][$col_ind])
break;
// If saddle point is present in this row then print it
if ($k == $n)
{
echo "Value of Saddle Point is: " .$min_row;
exit (0);
}
}
echo "No Saddle Point";
// If Saddle Point not found
?>
</body>
</html>
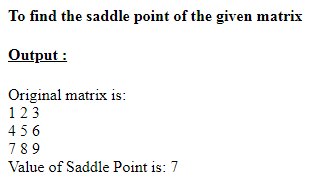
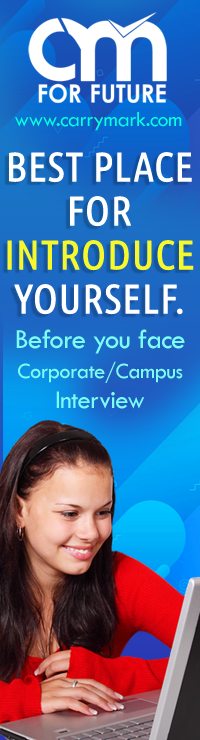