140. PHP Program to check given matrix is Magic Square or not.
How does this program work?
- In this program we are going to learn about how to check whether the given matrix is Magic Squre or not using PHP.
- In this program we calculated row sum, column sum and diagonal sum of distinct elements of the given matrix.
- If these sums are equal then print given matrix is magic square otherwise it is not a magic square matrix.
Here is the code
<html>
<head>
<title>PHP Program To check given matrix is magic square or not </title>
</head>
<body>
<?php
//Initialization of A matrix
$a =array
(
array(2, 7, 6),
array(9, 5, 1),
array(4, 3, 8)
);
$n = count($a);
// To print Original matrix
echo("Original matrix is: <br>");
for($i = 0; $i < $n; $i++) {
for($j = 0; $j < $n; $j++) {
echo ($a[$i][$j] . " ");
}
echo("<br>");
}
// Function to perform if matrix is magic square returns true else returns false
function MagicSquare($a)
{
$n = count($a);
$sum = 0; // To calculate the sum of the diagonal
for($i = 0; $i < $n; $i++)
$sum = $sum + $a[$i][$i];
// The secondary diagonal
$sum1 = 0;
for($i = 0; $i < $n; $i++)
$sum1 = $sum1 + $a[$i][$n-$i-1];
if( $sum != $sum1)
return false;
// To calculate the sums of Rows
for($i = 0; $i < $n; $i++)
{
$rowSum = 0;
for ($j = 0; $j < $n; $j++)
$rowSum += $a[$i][$j];
// To check if every row sum is equal to diagonal sum
if ($rowSum != $sum)
return false;
}
// To calculate the sums of Columns
for ($i = 0; $i < $n; $i++)
{
$colSum = 0;
for ($j = 0; $j < $n; $j++)
$colSum += $a[$j][$i];
// To check if every column sum is equal to diagonal sum
if ($sum != $colSum)
return false;
}
echo "Diagonal sum is: ".$sum."<br>";
echo "Secondary diagonal sum is: ".$sum1."<br>";
echo "Row sum is: ".$rowSum."<br>";
echo "Column sum is: ".$colSum."<br>"."<br>";
return true;
}
if (MagicSquare($a))
echo "Given matrix is Magic Square";
else
echo "Given matrix is not a magic Square";
return 0;
?>
</body>
</html>
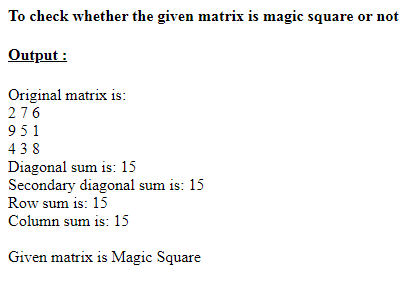
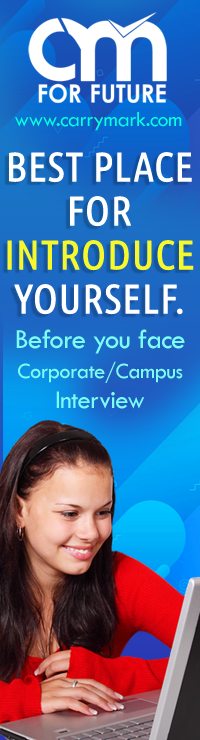