139. PHP Program to Transverse the given matrix helically.
How does this program work?
- In this program we are going to learn about how to transverse the given matrix helically using PHP.
- Elements are stored in array variable.
Here is the code
<html>
<head>
<title>PHP Program To transverse the given matrix helically</title>
</head>
<body>
<?php
$R = 3;
$C = 3;
function spiralPrint($m, $n, &$a)
{
$k = 0;
$l = 0;
// $k - starting row index $m - ending row index $l - starting column index $n - ending column index $i - iterator //
while ($k < $m && $l < $n)
{
// Print the first row from the remaining rows
for ($i = $l; $i < $n; ++$i)
{
echo $a[$k][$i] . " ";
}
$k++;
// Print the last column from the remaining columns
for ($i = $k; $i < $m; ++$i)
{
echo $a[$i][$n - 1] . " ";
}
$n--;
// Print the last row from the remaining rows
if ($k < $m)
{
for ($i = $n - 1; $i >= $l; --$i)
{
echo $a[$m - 1][$i] . " ";
}
$m--;
}
// Print the first column from the remaining columns
if ($l < $n)
{
for ($i = $m - 1; $i >= $k; --$i)
{
echo $a[$i][$l] . " ";
}
$l++;
}
}
}
echo " Traverse the matrix helically is: "."<br>"; $a = array(array(1, 2, 3),
array(4, 5, 6),
array(7, 8, 9));
spiralPrint($R, $C, $a);
?>
</body>
</html>
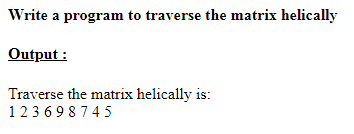
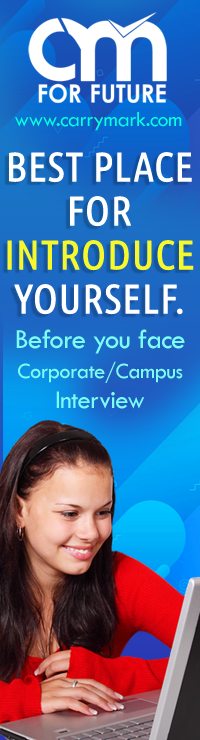