161. PHP Program to print name which occured more than once.
How does this program work?
- This program is used to print name which occured more than one time using PHP.
- String Palindrome means if it remains same even after reversing the each character.
- A string said to be a palindrome if original string is equal to the reverse of the given string otherwise it is not a palindrome.
Here is the code
<html>
<head>
<title>PHP Program To print name which occured more than one time</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="name" value="" placeholder="Enter a string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit'])) {
$string = $_POST['name'];
//Converts the string into lowercase
$string = strtolower($string);
//Split the string into words using built-in function
$words = explode(" ", $string);
// To print Original string
echo "Original string: "."</br>";
echo $string."</br>";
echo("Duplicate words in a given string :</br>");
for($i = 0; $i < count($words); $i++) {
$count = 1;
for($j = $i+1; $j < count($words); $j++) {
if( $words[$i] == $words[$j]) {
$count++;
//Set words[j] to 0 to avoid printing visited word
$words[$j] = "0";
}
}
//Displays the duplicate word if count is greater than 1
if($count > 1 && $words[$i] != "0")
{
echo ($words[$i]);
echo ("</br>");
}
}
return 0;
}
?>
</body>
</html>
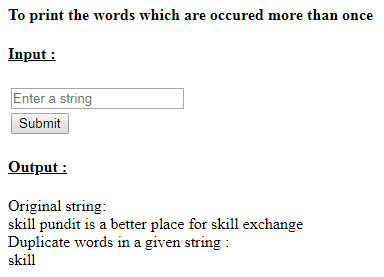
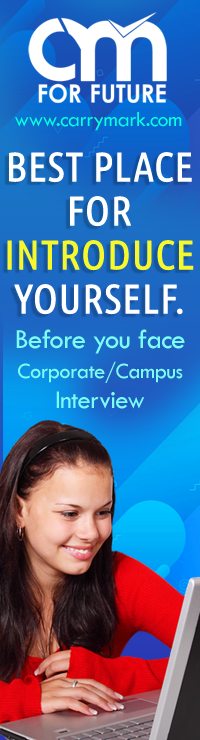