160. PHP Program to Check given String is Palindrome or Not.
How does this program work?
- This program is used to check given string is palindrome or not using PHP.
- Palindrome String is a string which means if it remains same even after reversing the given string.
- A string said to be a palindrome if original string is equal to the reverse of the given string otherwise it is not a palindrome.
Here is the code
<html>
<head>
<title>PHP Program To check given string is palindrome or not</title>
</head>
<body>
<form method="post">
<table border="0">
<tr>
<td> <input type="text" name="string" value="" placeholder="Enter a string"/> </td>
</tr>
<tr>
<td> <input type="submit" name="submit" value="Submit"/> </td>
</tr>
</table>
</form>
<?php
if(isset($_POST['submit'])) {
$a = $_POST['string'];
$b = strrev($a);
//To calculate reverse of the given string
$string_reverse = str_split($b);
$palin = '';
// To read string
foreach($string_reverse as $value)
{
$palin = $value;
}
//To compare given string and reverse string
if($a == $palin)
{
echo "Given String ".$a." is Palindrome";
}
else
{
echo "Given String ".$a." is not a Palindrome";
}
return 0;
}
?>
</body>
</html>
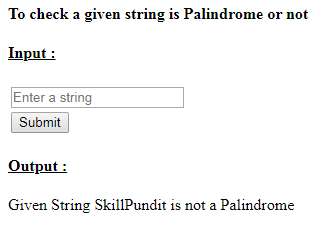
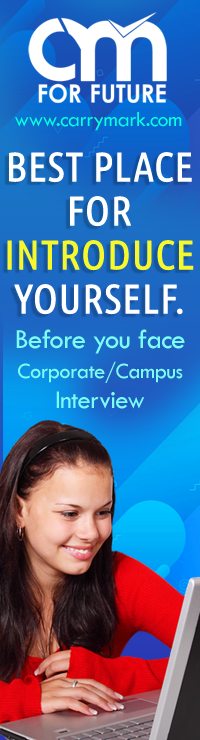