38. C Program to find Biggest of two numbers using switch case.
How does this program work?
- This code used to find biggest of two numbers.
- Here we take two variables to store two numbers.
- Here we compare the two numbers using swich case.
Here is the code
#include <stdio.h>
int main(void)
{
int num1, num2;
printf("Enter two numbers to find maximum: \n");
scanf("%d%d", &num1, &num2);
//If Expression (num1 > num2) will return either 0 or 1
switch(num1 > num2)
{
//If condition (num1>num2) is false
case 0:
printf("%d is Biggest", num2);
break;
// If condition (num1>num2) is true
case 1:
printf("%d is Biggest", num1);
break;
} return 0;
}
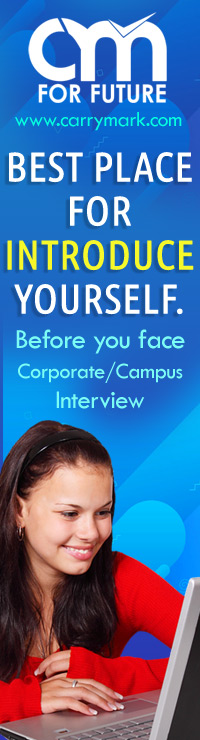