C - Language Theory
String
1. Strings are array of characters i.e. they are characters arranged one after another in memory. Thus, a character array is called string.
2. Each character within the string is stored within one element of the array successively.
3. A string is always terminated by a null character (i.e. slash zero 0).
You can see how to
- Compare Two Strings
- Concatenate Strings
- Copy one string to another string.
- String Length & perform Various string manipulation operations.
(You can perform such operations using the pre-defined functions of “string.h” header file. In order to use these string functions you must include string.h file in your C program)
You can use the gets() function to read the line of string and can also use puts() to display the string.
String Declaration
- char a[0] = {'S', 'K', 'I', 'L', 'L', 'P', 'U', 'N', 'D', 'I', 'T', '\0'};
- char a[0] = "Skillpundit";
Index | 0 | 1 | 2 | 3 | 4 | 5 |
---|---|---|---|---|---|---|
Variable | H | e | l | l | 0 | \0 |
Address | 0x23451 | 0x23452 | 0x23453 | 0x23454 | 0x23455 | 0x23456 |
Actually, you do not place the null character at the end of a string constant. The C compiler automatically places the '\0' at the end of the string. when it initializes the array. Let us try to print above mentioned string:
"C String tutorial"
c | s | t | r | i | n | g | t | u | t | o | r | i | a | l | \0 |
---|
strcat | Concatenate two strings |
strcmp | Compare two strings |
strcpy | Copy a string |
strlen | Get string length |
strlwr | To get the String Lower Case |
strupr | To get the String Upper Case |
strrev | To get the string reverse |
Purpose of Function
Function | Purpose |
---|---|
Strlen() | This function is used for finding a length of a string. It returns how many characters are present in a string excluding the NULL character. |
strcat(str1, str2) | This function is used for combining two strings together to form a single string. It Appends or concatenates str2 to the end of str1 and returns a pointer to str1. |
strcmp(str1, str2) | This function is used to compare two strings with each other. It returns 0 if str1 is equal to str2, less than 0 if str1 < str2, and greater than 0 if str1 > str2. |
strncmp(str1, str2) | It returns 0 if the first n characters of str1 is equal to the first n characters of str2, less than 0 if str1 < str2, and greater than 0 if str1 > str2. |
strlwr() | This function is used to convert string to lower case. |
strupr() | This function is used to convert string to upper case. |
strrev() | This function is used to reverse string of the original string. |
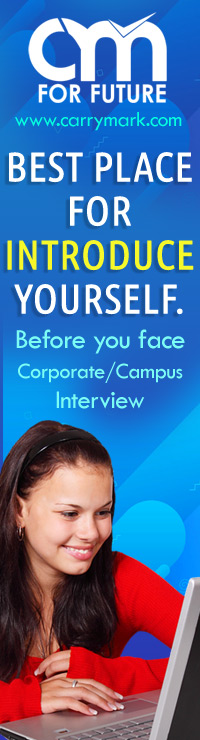