C - Language Theory
Operators:
An operator is a symbol that operates on a value or a variable.
For example: + is an operator to perform addition.
Types of operators in C :
- Arithmetic Operators
- Increment and Decrement Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Bitwise Operators
- Comma Operator
Arithmetic Operators
An arithmetic operator performs mathematical operations such as addition, subtraction, multiplication, and division etc on numerical values.
Operator | Meaning of Operator |
---|---|
+ | addition or unary plus |
- | subtraction or unary minus |
* | multiplication |
/ | division |
% | remainder after division (modulo division) |
Increment and Decrement Operators
C programming has two operators such as increment '++' and decrement '--' to change the value of an operand by 1.
Increment '++' means increases the value by 1 whereas decrement '--' means decreases the value by 1.
Assignment Operators
An assignment operator is used fpr assogmomg a value to a variable. The most common assignment operator is ' = '.
Operator | Example |
---|---|
= | a = b a = b |
+= | a += b a = a+b |
-= | a -= b a = a-b |
*= | a *= b a = a*b |
/= | a /= b a = a/b |
%= | a %= b a = a%b |
Relational Operators
A relational operator checks the relationship between two operands. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Operator | Meaning of Operator | Example |
---|---|---|
== | Equal to | (A && B) is false. |
> | Greater than | 5 > 3 is evaluated to 1 |
< | Less than | 5 < 3 is evaluated to 0 |
!= | Not equal to | 5 != 3 is evaluated to 1 |
>= | Greater than or equal to | 5 >= 3 is evaluated to 1 |
<= | Less than or equal to | 5 <= 3 is evaluated to 0 |
Logical Operators
An expression containing logical operators returns either 0 or 1 depending upon whether expression results true or false. If the relation is true, it returns 1; if the relation is false, it returns value 0.
Operator | Meaning of Operator | Example |
---|---|---|
&& | Logical AND. True only if all operands are true | If c = 5 and d = 2 then, expression ((c==5) && (d>5)) equals to 0. |
|| | Logical OR. True only if either one operand is true | If c = 5 and d = 2 then, expression ((c==5) || (d>5)) equals to 1. |
! | Logical NOT. True only if the operand is 0 | If c = 5 then, expression !(c==5) equals to 0. |
Bitwise Operators
During computation, mathematical operations like: addition, subtraction, multiplication, division, etc are converted to bit-level which makes processing faster and saves power.
Bitwise operators are used in C programming to perform bit-level operations.
Operator | Meaning of Operator |
---|---|
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise exclusive OR |
~ | Bitwise complement |
<< | Shift left |
>> | Shift right |
Comma Operator
Comma operators are used to link related expressions together.
Ex: int a, c = 5, d;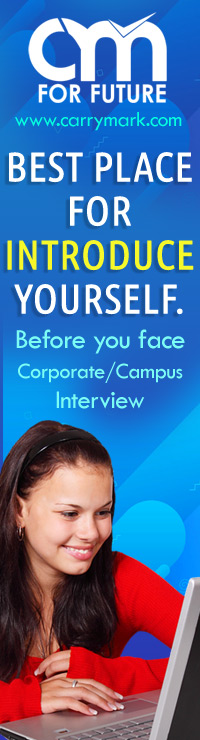