C program to display all possible permutations of letters of a string.
How does this program work?
- This Program is used to permutations of letters of a string in C.
Here is the code
#include <stdio.h>
#include <string.h>
void main()
{
char str[10];
int n;
printf("Enter String/Number\n");
scanf("%s",str);
n = strlen(str);
printf("All Possible Permutations:\n");
perm(str, 0, n - 1);
}
void perm(char *s, int a, int n)
{
int j;
if (a == n)
printf("%s\n", s);
else
{
for (j = a; j <= n; j++)
{
swap((s + a),(s + j));
perm(s, a+1, n);
swap((s + a), (s + j));
}
}
}
void swap(char* x, char* y)
{
char temp;
temp = *x;
*x = *y;
*y = temp;
}
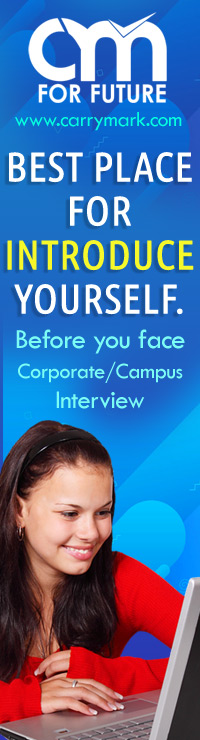