C - Language Theory
Variables
Variables are name of the memory location. It is used to store data.
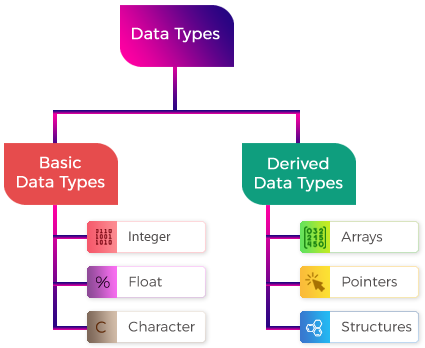
Basic Data Types:-
- Int: It is used to hold an integer value.
- float: It is used to hold float value.
- double: It is used to hold a double value.
- Char: It holds a character in it.
Size and Range of Data Types
Data types | Size in Bytes | Range | Format |
---|---|---|---|
Signed Char | 1 | -128 to 127 | %c |
Unsigned Char | 1 | 0 to 255 | %c |
Short Signed Int | 2 | -32768 to -32767 | %d |
Short Unsigned Int | 2 | 0 to 65535 | %u |
Signed Int | 2 | -32768 to -32767 | %d |
Unsigned Int | 2 | 0 to 65535 | %u |
Long Signed Int | 4 | -2147483648 to +2147483647 | %Ld |
Long Unsigned Int | 4 | 0 to 4294967295 | %Lu |
Float | 4 | 3.4e-38 to 3.4e+38 | %f |
Double | 8 | 1.7e-308 to 1.7e+308 | %Lf |
Long Double | 10 | 3.4e-4932 to 1.1e+4932 | %LF |
Rules for defining variable
- Variable type can be char, int, float, double.
- A variable name can consist of alphabets, digits and the underscore character.
- Variable name can start with alphabet, and underscore. It can not start with a digit.
- Blank spaces cannot be used in variable names.
- Special characters like $, # are not allowed.
- Keywords are not allowed as variable name, i.e int, float, char, etc.
Local Variable
A variable that is declared inside the function or block is called a local variable.
Syntax |
---|
Void function1() { int x=5;//local variable } |
Global Variable
A variable that is declared outside the function or block is called a global variable.
Syntax |
---|
{
int a=10;//global variable } |
Static Variable
A variable that is declared with the static keyword is called static variable. It retains its value between multiple function calls.
Syntax |
---|
void function1() { int x=5;//local variable static int y=5;//static variable x=x+1 y=y+1 printf ("%d,%d",x,y); } |
Note: if you call the function many times, the local variable prints the same value in each function ie 6, 6, 6 and so on. But in static variable prints the increment value in each function i.e 6, 7, 8 and so on.
Automatic Variable
All variables in C that are declared inside the block. are automatic variables by default. We can explicitly declare an automatic variable using auto keyword.
Syntax |
---|
void function1() { int x=5;//local variable (also automatic) auto int y=6;// automatic variable } |
External Variable
We can share a variable in multiple C source files by using an external variable. To declare an external variable. you need to use extern keyword.
Syntax |
---|
#include<stdio.h> void print() { extern int g = 10; printf ("g=%d\n",g); } int main() { g = 7; printf ("g=%d\n",g); printf (); return 0; } |
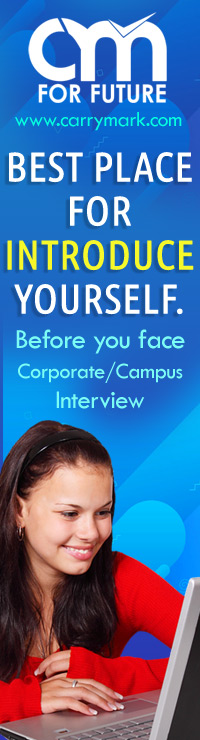