127. C Program to Compute the 1-x2/(2^21!) + x4/(2^4*2!) – x6/(2^6*3!) series.
How does this program work?
- This program is used to compute the sum of series for the given order.
- Here we are going to find the series for 1-x2/(2^21!) + x4/(2^4*2!) – x6/(2^6*3!) upto nth term.
- where The nth term is the user input.
- Computing the given series is easy by Using the simple for loop condition.
Here is the code
#include <stdio.h>
#include <math.h>
double Series(double x, int n)
{
double sum = 1, term = 1, fct = 1, p =1, multi = 1;
// Computing sum of remaining n-1 terms.
for (int i = 1; i < n; i++)
{
fct = fct * multi * (multi+1);
p = p*x*x;
term = (-1) * term;
multi += 2;
sum = sum + (term * p)/fct;
}
return sum;
}
// Input values
int main()
{
double x = 9;
int n = 10;
printf("%.4f", Series(x, n));
return 0;
}
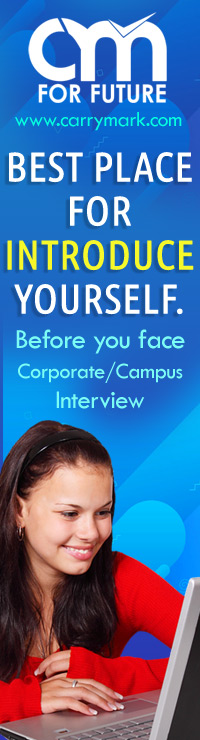