77. C Program to Convert Binary Value into Decimal Number.
How does this program work?
- This Program is used to convert Binary value into decimal number using C program.
- Here we need only input interms of 0 and 1s. and it should not be more than 5 digits.
- decimal number means it is the base of 10.
- The below code convert base of 2 to base of 10.
Here is the code
#include <stdio.h>
int main(void)
{
int num, binary_val, decimal_val = 0, base = 1, rem;
printf("Enter a binary number(1s and 0s) \n");
scanf("%d", &num);
//Maximum five digits
binary_val = num;
while (num > 0)
{
rem = num % 10;
decimal_val = decimal_val + rem * base;
num = num / 10 ;
base = base * 2;
}
printf("The Binary number is = %d \n", binary_val);
printf("Its decimal equivalent is = %d \n", decimal_val);
return 0;
}
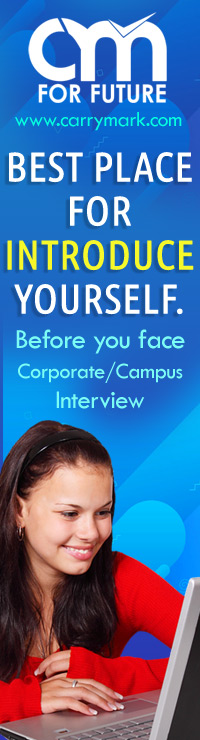