79. C Program to Convert Decimal number Into hexadecimal Number.
How does this program work?
- This Program is used to convert Decimal number into hexadecimal number using C program.
- Decimal number means it is a simple integers 0 to 9.
- Hexa decimal contains 0 to 9 numbers and in addition with A, B, C, D, E, F. So totally they are 16 numbers.
- For every 16 numbers only multiples of 10 will come.
- This below code explains how to convert a decimal integer into hexadecimal.
Here is the code
#include <stdio.h>
int main(void)
{
long num, quotient, r;
int i, j = 0;
char hexadecimalnum[100];
printf("Enter decimal number: \n");
scanf("%ld", &num);
quotient = num;
while(quotient != 0)
{
r = quotient % 16;
if(r < 10)
hexadecimalnum[j++] =48 + r;
else
hexadecimalnum[j++] = 55 + r;
quotient = quotient / 16;
}
// Display integer into character
printf("Equivalent Hexa value of decimal is");
for(i = j; i >= 0; i-- )
printf("%c", hexadecimalnum[i] );
return 0;
}
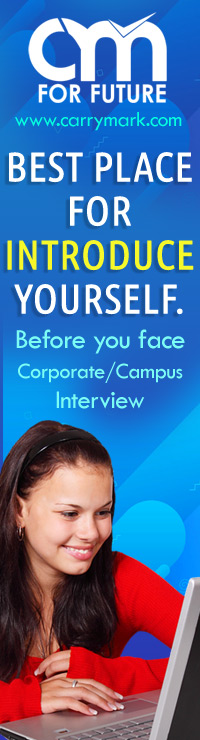