200. C Program to find total number of characters in a string.
How does this program work?
- This Program is used to find total number of characters in a string.
- Define and initialize a variable count to 0.
- Iterate through the string till the end and for each character except spaces, increment the count by 1.
- To avoid counting the spaces check the condition i.e. string[i] != ' '.
Here is the code
#include <stdio.h>
#include <string.h>
int main(void)
{
char str[50];
int i = 0, count = 0;
printf("\nEnter Your String: ");
gets(str);
for(i=0; i < strlen(str); i++)
{
if ( str[i] != ' ')
{
count++;
}
}
printf("\nNumber of characters: %d", count);
return 0;
}
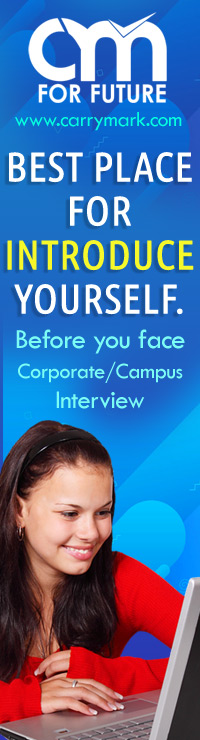