15. C Program to find the Distance between Two Points.
How does this program work?
- In this programme you will learn about how to measure the distance between two points using x,y Coordinates.
- first we need to store user entered values in x1,y1 and x2,y2.
- After that by using the given formula we can get the distance between two points using the below c program.
Here is the code
#include <stdio.h>
#include <math.h>
int main(void)
{
int x1,x2,y1,y2;
int x,y;//Temporary variables
float distance;
printf("Enter X and Y coordinates of first point\n");
scanf("%d %d",&x1,&y1);
printf("Enter X and Y coordinates of Second point\n");
scanf("%d %d",&x2,&y2);
x=x2-x1;
y=y2-y1;
distance=sqrt((x*x)+(y*y));//To compute the distance
printf("Distance: %.2f\n",distance);
return 0;
}
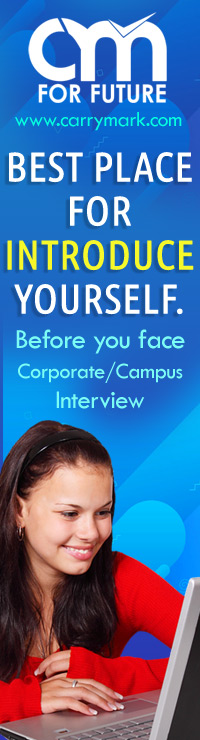