145. C Program to find Factorials of the Given Number using Recursion.
How does this program work?
- In this C programme you will learn about how to find the factorials of the given number.
- Here recursion method is used to get the output.
Here is the code
#include <stdio.h>
int getfactorial(int);
int main()
{
int num, fact;
//User input
printf("Enter a number to find factorial:\n");
scanf("%d",&num);
fact =getfactorial(num);
//Display the input number
printf("\nfactorial of %d is: %d",num, fact);
return 0;
}
int getfactorial(int n)
{
//Factorial of 0 is 1
if(n==0)
return(1);
//Function calling itself: recursion
return(n*getfactorial(n-1));
}
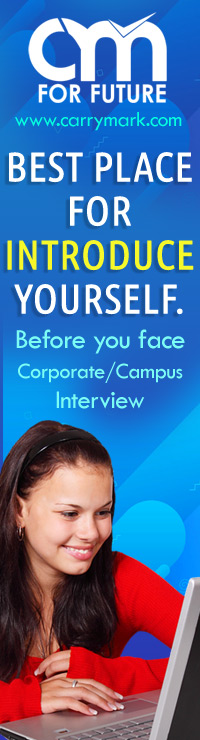