68. C Program To find GCD of Given N numbers.
How does this program work?
- This Program is used to find the GCD of a given n numbers.
- Here we used arrays to store the elements in sequence.
- By using the for loop and if condition G.C.D we get the desired LCM.
Here is the code
#include <stdio.h>
int main(void)
{
//Initialize variables
int n,i,gcd;
printf("Enter Size of numbers : \n");
scanf("%d",&n);
int arr[n];
printf("\nEnter numbers :- \n ");
for(i=0;i< n;i++)
{
printf("Enter your %d number = \n",i+1);
scanf("%d",&arr[i]);
}
gcd=arr[0];
int j=1;
while(j < n)
{
if(arr[j]%gcd==0)
{
j++;
}
else
{
gcd=arr[j]%gcd;
i++;
}
}
printf("GCD of N no.s = %d \n",gcd);
return 0;
}
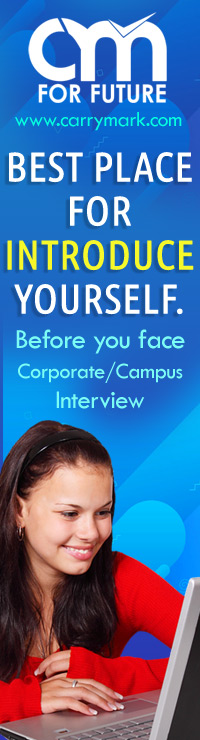