70. C Program To find Second Biggest From the given Array.
How does this program work?
- This Program is used to find second biggest and second smallest numbers from the given array.
Here is the code
#include <stdio.h>
#include <limits.h> //To define Maximum value limit
int main()
{
int arr[50], i, Size;
int first, second;
//Initialize the size of array
printf("\n Please Enter the Number of elements in an array : ");
scanf("%d", &Size);
//To store the user inputs
printf("\n Please Enter %d elements of an Array \n", Size);
for (i = 0; i < Size; i++)
{
scanf("%d", &arr[i]);
}
first = second = INT_MIN;
//computes the second largest Number in array
for (i = 0; i < Size; i++)
{
if(arr[i] > first)
{
second = first;
first = arr[i];
}
else
if(arr[i] > second && arr[i] < first)
{
second = arr[i];
}
}
printf("\n The Second Largest Number in this Array = %d", second);
return 0;
}
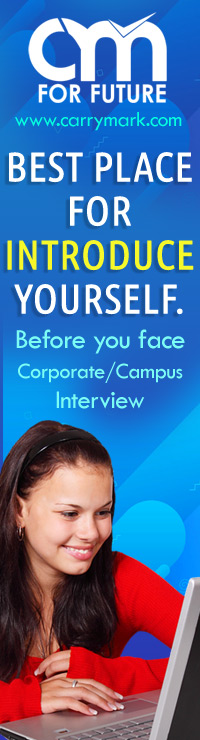