148. C Program to find the Sum of n natural numbers using recursion.
How does this program work?
- In this C programme you will learn about how to find the sum of n natural numbers using recursion.
Here is the code
#include <stdio.h>
int sum(int a)
{
if(a == 0)
return 0;
return (a + sum(a-1));//Compute sum
}
int main()
{
int a; //Intialise the varible
printf("Enter a number :\n" );
scanf("%d", &a);
printf("Sum of %d Natural Numbers is %d\n ",a,sum(a));
return 0;
}
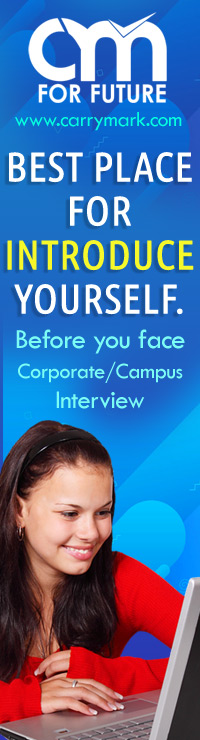