131. C Program to Multiplication of Two Matrices.
How does this program work?
- In this programme you will learn about how to Multiply two matrix using C Language.
- variables are stored in two arrays.
- And then by using the Multiplication process result is find.
Here is the code
#include <stdio.h>
int main(void)
{
int a[10][10], b[10][10], result[10][10], row1, col1, row2, col2, i, j, k;
printf("Enter rows and column for first matrix: ");
scanf("%d %d", &row1, &col1 );
printf("Enter rows and column for second matrix: ");
scanf("%d %d",&row2, &col2 );
// Column of first matrix should be equal to column of second matrix and
while (col1 != row2)
{
printf("Error! column of first matrix not equal to row of second.\n\n");
printf("Enter rows and column for first matrix:");
scanf("%d %d", &row1, &col1 );
printf("Enter rows and column for second matrix");
scanf("%d %d",&row2, &col2 );
}
// Storing elements of first matrix
printf("\nEnter elements of matrix 1:\n");
for(i=0; i< row1; ++i)
for(j=0; j< col1; ++j)
{
printf("Enter elements a%d%d: ",i+1, j+1);
scanf("%d", &a[i][j] );
}
// Storing elements of second matrix
printf("\nEnter elements of matrix 2:\n");
for(i=0; i< row2; ++i)
for(j=0; j< col2; ++j)
{
printf("Enter elements b%d%d: ",i+1, j+1 );
scanf("%d",&b[i][j] );
}
// Initializing all elements of result matrix to 0
for(i=0; i< row1; ++i)
for(j=0; j< col2; ++j)
{
result[i][j] = 0;
}
// Multiplying matrices a and b and
// Storing result in result matrix
for(i=0; i< row1; ++i)
for(j=0; j< col2; ++j)
for(k=0; k< col1; ++k)
{
result[i][j]+=a[i][k]*b[k][j];
}
// Displaying the result
printf("\nOutput Matrix:\n");
printf("\nMultiplication of Two Matrix:\n");
for(i=0; i< row1; ++i)
for(j=0; j< col2; ++j)
{
printf("%d ", result[i][j]);
if(j == col2-1)
printf("\n\n");
}
return 0;
}
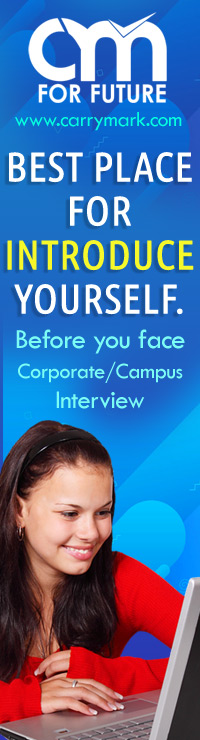