42. C Program to check number existing given array or not.
How does this program work?
- In this program your going to learn about how to check number existing given array or not.
Here is the code
#include <stdio.h>
#define Max_Size 100 // Array size is defined
int main()
{
//To check whether an element is exit in array or not
int arr[Max_Size];
int size, i, Search, found;
//Enter Input size of array
printf("Enter size of array: \n");
scanf("%d", &size);
// Enter Input elements of array
printf("Enter elements in array: \n");
for(i=0; i < size; i++)
{
scanf("%d", &arr[i]);
}
printf("Enter element to search: \n");
scanf("%d", &Search);
//Take a dummy value 0
found =0;
for(i = 0; i < size; i++)
{
// If the element is found in array then raise found =1 and terminate from loop.
if(arr[i] == Search)
{
found = 1;
break;
}
}
// If the element is not found in array
if(found == 1)
{
printf("%d is found at position %d\n", Search, i + 1);
}
else
{
printf("%d is not found in the array\n", Search);
}
return 0;
}
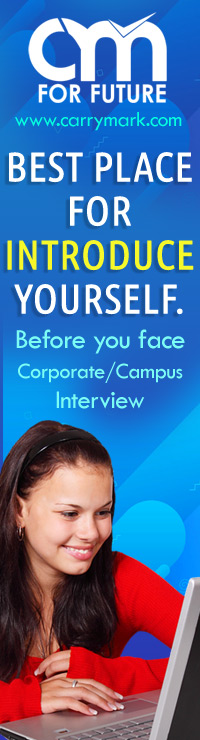