180. C Program to Find Neither Big Nor Small in Given 3numbers using Pointers.
How does this program work?
- This Program is used to find Neither big nor small number from given 3numbers using pointers.
Here is the code
int main()
{
int num1, num2, num3;
int *p1, *p2, *p3;
//Taking input from user
printf("Enter First Number: \n");
scanf("%d",&num1);
printf("Enter Second Number: \n");
scanf("%d",&num2);
printf("Enter Third Number: \n");
scanf("%d",&num3);
//Assigning the address of input numbers to pointers
p1 = & num1;
p2 = & num2;
p3 = & num3;
if(*p2>*p1 && *p1>*p3 || *p3>*p1 && *p1>*p2)
{
printf("\n%d is a middle number\n", *p1);
}
else //Checking num2 is a middle number or not
if(*p1>*p2 && *p1>*p3 || *p3>*p2 && *p2>*p1)
{
printf("\n%d is a middle number\n",*p2);
//Checking num3 is a middle number or not
}
else if(*p1>*p3 && *p3>*p2 || *p2>*p3 && *p3>*p1)
{
printf("\n%d is a middle number\n",*p3);
}
return 0;
}
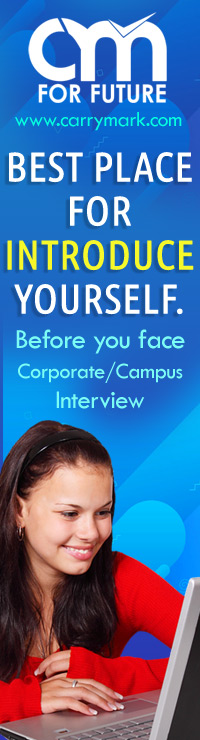